Client Analytics
Base Global‑e Script
The Global‑e base script file must be included in all Merchant pages (Layout/Master).
You can host the globale.merchant.client.js
JavaScript file locally or it can be served from the Global-e servers, see here.
https://<domain>/merchant/clientsdk/<merchant id>?v=<version>
To load our base script, we recommend that you use a self-invoking function.
(<window>, <document>, <sDomain> + <clientJsURL>, "script", "gle", <scriptVersion>, <merchantId>)
Production Example:
(window, document, "https://web.global-e.com" + "/merchant/clientsdk/124125?v=2.1.4", "script", "gle", "2.1.4", 124125)
Sample
<script> //Global‑e script initializer (function (w, d, u, t, o, h, m, s, l) { w["globaleObject"] = o; w[o] = w[o] || function () { (w[o].q = w[o].q || []).push(arguments) }; w[o].m = m, w[o].v = h; s = d.createElement(t); l = d.getElementsByTagName(t)[0]; s.async = true; s.src = u + "?v=" + h; l.parentNode.insertBefore(s, l); })(<window>, <document>, <globaleaddon.clientJsDomain> + <globaleaddon.clientJsUrl>, "script", "gle", <globaleaddon.scriptVersion>, <globaleaddon.merchantId>); </script>
Script Parameters
Parameter | Description |
---|---|
| Production: https://web.global-e.com Integration: https://www.bglobale.com Staging: https://www2.bglobale.com |
| The JavaScript window object |
| The JavaScript document object |
| Local file path or direct to Global‑e servers /merchant/clientsdk/<merchantId>?v=<scriptVersion> |
| The version of Global-e server API. Currently 2.1.4. |
| The current Merchant ID |
This script loads the Global-e client script asynchronously.
The Global‑e client script uses an asynchronous approach so that you can start calling actions immediately; these actions are executed once the script is fully loaded.
Note
This list is subject to modifications and limitations that may affect the availability of payment methods on the checkout page.
Order Transaction Analytics
The Global-e Checkout Analytics feature enables the merchant (you) to obtain and track customer activity and use this information for analytics. This feature is part of the Global-e Client SDK.
This document focuses on the implementation of the Global-e Client SDK Order Transaction Analytics event; it targets teams in charge of implementing analytics, trackers, and other front-end implementations related to checkout events.
The Global-e Client JavaScript SDK implements the front-end UX features, (for example, Country and Currency switching) and integration (analytics) features on your website.
You can use the Client SDK to gather data and integrate the checkout information with any analytics framework, such as Google Analytics, or with any pixel type or tracker solution.
The following figure provides an overview of the Global-e Client SDK subscription flow.
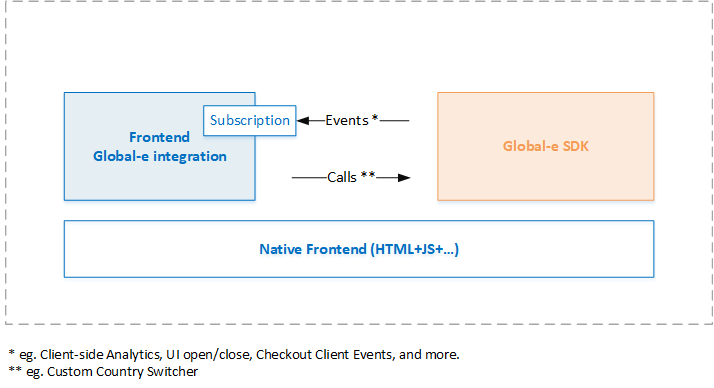
The building blocks of the Checkout Analytics subscription flow are as follows:
The merchant subscribes to the Global-e SDK Events (callback).
When specific actions are performed by the customer on the Merchant's website, the Global-e Client SDK sends a trigger, and the event returns the relevant information in JSON format.
To implement analytics, use the Global-e Client SDK OnCheckoutStepLoaded
event functionality, as detailed in this document.
The following figure provides an overview of the Global-e Checkout analytics flow.
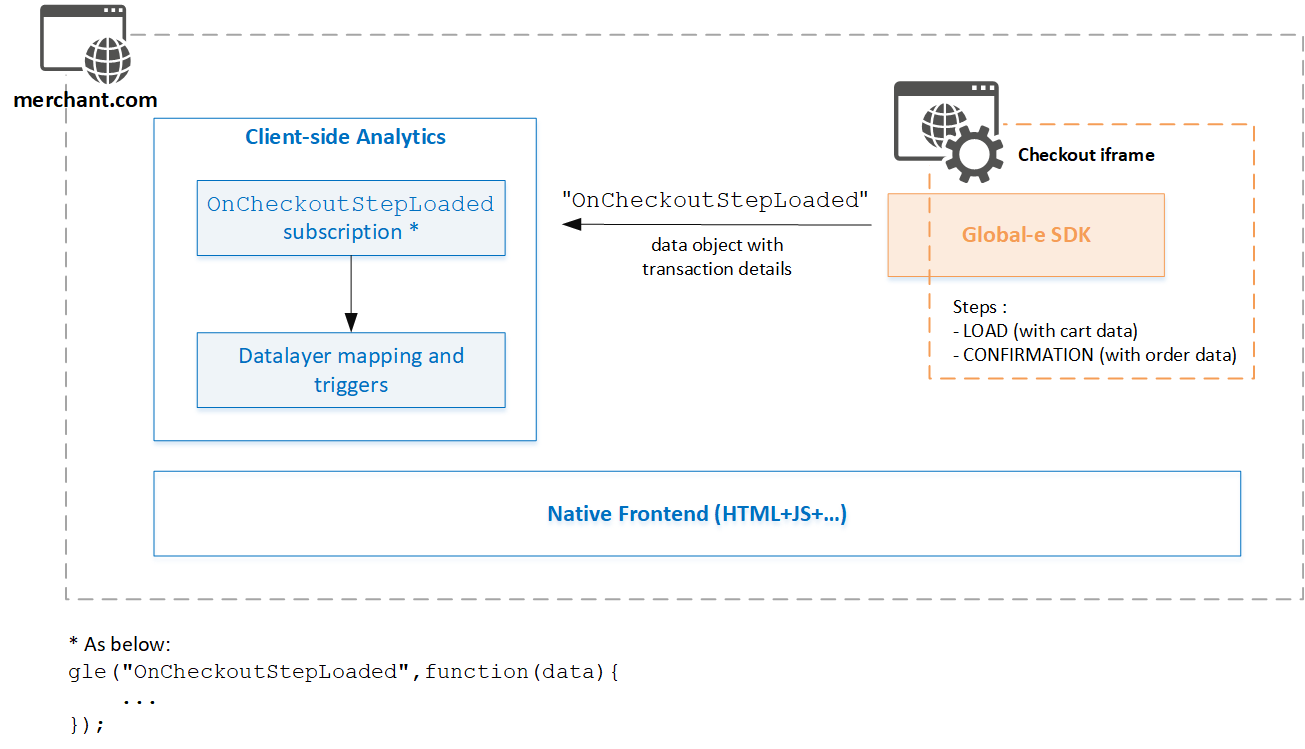
The building blocks of the Global-e Checkout analytics subscription flow are as follows:
Subscribe to the Global-e
OnCheckoutStepLoaded
callback, as described in Subscribing to OnCheckoutStepLoaded.After subscription, when the Global-e
OnCheckoutStepLoaded
is triggered based on checkout activities (LOAD
orCONFIRMATION
), the Global-e Checkout iFrame sends you back checkout and order information in JSON format.Map the checkout data returned by the
OnCheckoutStepLoaded
to your existing analytics implementation so that you can create conversion reports in your analytics dashboard.Note
Ad-blockers and payment redirects can affect analytics reporting.
The responsibilities between Global-e and the Merchant shall be shared as follows:
Global-e shall provide and maintain the Global-e Order Transaction object, as specified by Global-e. In addition, Global-e shall provide the support required to assist the Merchant with their implementation efforts.
The Merchant shall implement the subscription, data layer mapping, and Quality Assurance testing.
Make sure to exclude web.global-e.com and webservices.global-e.com as referrals (as per https://support.google.com/analytics/answer/2795830?hl=en)
OnCheckoutStepLoaded
This section describes how to subscribe to the OnCheckoutStepLoaded
callback, the functionality of the callback and the Transaction Analytics object, and how to implement this feature for analytics.
OnCheckoutStepLoaded
is triggered twice:
Checkout Page
LOAD
step:The LOADED step is called when the customer visits the checkout page or changes the destination country. Upon trigger, the Global-e Client sends the OnCheckoutStepLoaded event together with a subset of the Order Transaction Analytics data, enabling the merchant to get product and cart information.
Checkout
CONFIRMATION
step:When the checkout completes (Checkout Confirmation), the Global-e Client sends the OnCheckoutStepLoaded event again, but this time, together with the Order Transaction Analytics data. The Order Transaction Analytics contains product, and payment information, as well as transaction information between the customer and Global-e and reconciliation transaction information between Global-e and the merchant.
Global-e provides pre-built subscriptions for the platforms it supports. Subscription implementation may vary according to the platform. Refer to the relevant platform documentation for platform-specific instructions.
To subscribe to OnCheckoutStepLoaded:
You can use one of the following subscription options:
If not already included, incorporate the
OnCheckoutStepLoaded
subscription snippet directly into your Checkout page (only).Load the subscription from an external resource via tag manager (for example, Google Tag Manager).
Implement your required triggers and data layer around the checkout process.
The subscription to the OnCheckoutStepLoaded
callback is illustrated in the following snippet.
gle("OnCheckoutStepLoaded",function(data){...}
OnCheckoutStepLoaded Callback
This section provides a description of the OnCheckoutStepLoaded
callback, the data, and how the data is passed.
The OnCheckoutStepLoaded
is called twice, once for the initial checkout page load and the second time for the checkout confirmation page.
gle("OnCheckoutStepLoaded", function (data) { switch (data.StepId) { case data.Steps.LOADED: if (data.IsSuccess) { // data contains product and cart information; // Include analytics triggers here // use the data object fields; break; case data.Steps.CONFIRMATION: if (data.IsSuccess) { // data contains order information; // Include analytics triggers here // use the data object fields; // window.location = "http://www.yourconfirmationpage.com"; } break; } });
The following table details the OnCheckoutStepLoaded
callback properties.
Property | Description |
---|---|
| The Global-e SDK instance to subscribe to, gle or glegem, depending on Platform. |
| The name of the callback |
| Your implementation of the The callback function is called twice, once when the checkout starts and once when the checkout ends.
|
| The data parameter contains the object that holds the event data. The data structure elements are detailed below. |
data
{ "Steps": { "LOADED": 0 //"The checkout page (step) has loaded", "CONFIRMATION": 4 //"The confirmation page is displayed" }, //---------------Applicable to the Confirmation Step------------------ "OrderID": "Global‑e order ID", //Confirmation only "AuthCode": "Global‑e payment authorization code", //Confirmation only //-------------------------------------------------------------------- "StepId": "" //"The Global‑e step ID", "IsSuccess": "", "details": {
Where:
Property | Type | Description |
---|---|---|
| object (enum) | Checkout step and confirmation step Values: { |
| Integer | The checkout page (step) has loaded. Global-e checkout loaded code. See Data structure and examples. |
| Integer | The confirmation page (step) is displayed immediately after the payment has been authorised. Global-e confirmation code. See Data structure and examples. |
| Integer | The Global-e
|
| Object | This field can contain:
The Global-e Order Transaction Analytics Object contains all the order properties and e-commerce transactions, as detailed in the following sections. |
LOADED Step (StepId: 0)
When the LOADED
step is triggered, the data property includes the following Checkout Load Object populated with cart data.
The information included in the Loaded Step is a subset of the Confirmation step. Therefore, fields related to the order have an empty value.
Note
If the page is reloaded following a change of country, this object may also include Checkout information, such as customer billing and shipping details, as in the Transaction object (confirmation step).
{ "Steps": { "LOADED": 0 //"The checkout page (step) has loaded", "CONFIRMATION": 4 //"The confirmation page is displayed"" }, "IsSuccess": “true” //"The value is always true", "StepId": 0 //"The Global‑e step ID", "details": { "CustomerCurrencyCode": "", //See Order Properties "MerchantCurrencyCode": "", //See Order Properties "IsEU": "", //See Order Properties "CustomerDetails" " MerchantUserId": "" //See Customer Details "HubCountryCode": "", //See Order Properties "ProductInformation" : "", //See Product Information "Discounts" : "", //See Discounts "cultureCode": "", //See Order Properties "InitialCheckoutCultureCode": "", //See Order Properties "URL" : "", //See Global‑e Properties "CustomURL" : "", //See Global‑e Properties "MerchantID" : "", //See Global‑e Properties "Title" : "", //See Global‑e Properties "CustomHostName" : "", //See Global‑e Properties "PageType" : "", //See Global‑e Properties "CartToken" : "", //See Global‑e Properties "URLReferer" : "", //See Global‑e Properties "DoLog" : "" //See Global‑e Properties }, }
See:
The Order Transaction Analytics Object section and subsections for a description of the properties.
Checkout LOAD Step example.
CONFIRMATION Step (StepId: 4)
When the CONFIRMATION
step is triggered, the data property contains the following JSON object:
{ "Steps": { "LOADED": 0 //"The checkout page (step) has loaded", "CONFIRMATION": 4 //"The confirmation page is displayed" }, "IsSuccess": "Whether the payment was successful", "OrderID": "Global‑e order ID", "AuthCode": "Global‑e payment authorization code", "StepId": 4 //"The Global‑e step ID", "details": { // "Order Transaction Analytics Object" "OrderID": "", "CartToken": "", "CountryCode": "", "UrlReferer": "", //See Order Transaction Analytics Object section and subsections //for the complete list of properties and descriptions. } }
Where:
Property | Type | Description |
---|---|---|
| Bool | If true, the payment ( If the data returns |
| String | The Global‑e Order ID. Example: GE103411992GB |
| Integer | The Global‑e payment authorization code. Example: 5 |
See:
Order Transaction Analytics Object section and subsections for the complete list of properties and descriptions.
Examples for an Analytics implementation example and various order scenarios.
Order Transaction Analytics Object Overview
The "OnCheckoutStepLoaded data.details"
parameter contains the Order Transaction Analytics Object with a breakdown of general ordering information and transactions.
Structure
The Order Transaction Analytics data object consists of a list of data properties set up as groups and components, generally structured as detailed below.
Order Transaction Analytics Object Data Structure
{ // General information ... "CustomerDetails": { "MerchantUserId": "ShippingAddress": { ... }, "BillingAddress": { ... }, }, // Order Prices Component "OrderPrices": { // Merchant Transaction Group "MerchantTransaction": { ... }, // Merchant Transaction Exclusive of VAT Group "MerchantTransactionExcludingLocalVAT": { ... }, // Customer Transaction Group "CustomerTransaction": { ... }, // Customer Transaction In Merchant Currency Group "CustomerTransactionInMerchantCurrency": { ... }, }, // Product Information Component "ProductInformation": [{ ... "ProductPrices": { "MerchantTransaction": { ... }, "MerchantTransactionExcludingLocalVAT": { ... }, "CustomerTransaction": { ... }, "CustomerTransactionInMerchantCurrency": { ... }, } } ], // Payment Component "PaymentMethods": [{ ... }, ], // Discounts Component "Discounts": [{ ... "DiscountPrices": { "MerchantTransaction": { ... }, "MerchantTransactionExcludingLocalVAT": { ... }, "CustomerTransaction": { ... }, "CustomerTransactionInMerchantCurrency": { ... }, } } ] }
Data Groups
The Order Transaction Analytics data groups consist of ecommerce transactions containing information such as order details, prices, and discounts, listed in merchant and customer currencies.
Each time a customer places an order, there are two separate Global‑e transactions:
A transaction between Global-e and the merchant
A transaction between Global‑e and the customer
Transactions between the different parties are set up as data groups, as follows:
Note
VAT does not apply to the US. The VAT value is 0 for all non-EU merchants.
Merchant transaction: This group contains the prices and discounts in the merchant currency for the transaction between the merchant and Global‑e. It also includes the formulas for shipping cost estimations. These prices include VAT (between Global‑e and the merchant), if applicable.
All values other than the shipping values, match the reconciliation reports.
Merchant transaction excluding local VAT: This group contains all prices and discounts between the merchant and Global-e. Prices are listed in the merchant’s currency, excluding the VAT between Global-e and the merchant, if applicable.
Customer transaction: This group contains all prices and discounts listed in the customer’s currency, reflecting the price paid and seen by the customer on the checkout page.
Customer transaction in merchant currency: This group contains the price paid and seen by the customer in the checkout page converted to merchant currency. The conversion is based on the spot exchange rate.
Components
Object properties are grouped as components, as follows:
Order information: This component includes all order level details properties. See Order Prices Properties
Product information: This component includes all product-level information that does not include prices. See
Payment methods: This component lists the method used to make the payment, such as with a standard credit or debit card or with an alternative method (such as PayPal, for example). See Payment Methods
Discounts: This component lists the discount values applied to the order (cart or product level), according to the original list of discounts received in the cart information for this order, and to the Merchant shipping configuration on Global‑e. See Discount Information
Data Details Properties
This section details the Transaction Object's data, including order properties, product properties, order payment methods, and discount information.
Order Properties
Property | Type | Description |
---|---|---|
| String | Global‑e order unique identifier Example: |
| Bool | Indicates whether the order is a replacement. |
| String | 3-char ISO currency code denoting the customer’s currency. Example: |
| String | 3-char ISO currency code denoting the original (merchant) currency on the merchant’s side. Example: |
| Bool | Whether the destination country is in the EU. |
| - | See CustomerDetails section. |
| String | Two-letter country code of the hub from which the order is dispatched to the customer. Example: |
| String | Customer state or region (if available). |
| String | Merchant website or store URL. |
| String | Indicates if the customer has selected the “guaranteed duties and taxes” option. |
| String | The name of the carrier, as was defined by Global-e for the specific merchant. Example: DHL, Spring Parcel Over 2Kg |
| String | The type of carrier, as selected by the customer on the checkout page. Example: Standard, Express |
| Decimal | The minimum number of days to delivery, as displayed on the Checkout page. |
| Decimal | The maximum number of days to delivery, as displayed on the Checkout page. |
| String | Culture code (language). If specified, the textual properties will be returned in the requested culture’s language if available. Texts in English will be returned by default |
| String | Culture code determined on initial checkout load |
| CustomerDetails | The checkout step ( |
| String | Order destination country code 2-char ISO. Example: |
Customer Details
Note
'CustomerDetails' is disabled by default. Make sure to request that Global-e enables it.
MerchantUserId
Property | Type | Description |
---|---|---|
| String | The registered user ID as it figures on the merchant’s website Value for guests: “null” |
ShippingAddress
Property | Type | Description |
---|---|---|
| String | The first name of the customer's shipping address |
| String | The last name of the customer's shipping address |
| String | The first line of the customer’s shipping address |
| String | The second line of the customer's shipping address |
| String | The customer’s shipping city |
| String | The customer’s shipping country name |
| String | The customers shipping country code ISO-2 |
| String | The customer's shipping state name (if applicable) |
| String | The customer’s shipping state code ANSI (if applicable) |
| String | The customer’s shipping company name |
| String | The customer’s shipping zip code |
| String | The customer’s shipping phone number |
BillingAddress
Property | Type | Description |
---|---|---|
| String | The first name of the customer's billing address |
| String | The last name of the customer's billing address |
| String | The first line of the customer's billing address |
| String | The second line of the customer's billing address |
| String | The customer's billing city |
| String | The customer's billing country name |
| String | The customer's billing country code ISO-2 |
| String | The customer's billing state name (if applicable) |
| String | The customer's billing state code ANSI (if applicable) |
| String | The customer's billing company name |
| String | The customer's billing zip code |
| String | The customer's billing phone number |
| String | The customer's billing email address |
Order Prices Properties
This section details order-level merchant and customer transaction properties.
MerchantTransaction
This section details the order transactions between the merchant and Global-e.
Global-e reconciles with the merchant in the merchant transaction currency, as denoted in the MerchantCurrencyCode
property.
Property | Type | Description |
---|---|---|
| Decimal | Estimated total order price after deducting the shipping and duties discounts. |
| Decimal | Total price of the products (only) before discounts |
| Decimal | Total price of the products after product discounts |
| Decimal | The estimated shipping cost to be subsidized by the merchant |
| Decimal | Shipping service fee VAT rate, if applicable |
| Decimal | Duties subsidy discount |
| Decimal | The VAT paid by Global-e to the merchant on the products, if applicable. |
| Decimal | The total VAT sum to be paid by Global-e to the merchant on the order, if applicable. |
| Decimal | Merchant Remote Area Surcharge Subsidy This value is included in If this fee is not subsidized by merchants, this value is null. ( |
MerchantTransactionExcludingLocalVAT
This section details the order transactions between the merchant and Global-e excluding VAT.
Global-e reconciles with the merchant in the merchant transaction currency, as denoted in the MerchantCurrencyCode
property.
Property | Type | Description |
---|---|---|
| Decimal | Estimated total order price after deducting the discounts |
| Decimal | Total price of the products (only) before discounts |
| Decimal | Total products price after discounts |
| Decimal | The estimated shipping cost to be subsidized by the merchant |
| Decimal | The shipping price paid by the customer |
| Decimal | Duties subsidy discount taken in charge by the merchant |
| Decimal | Merchant Remote Area Surcharge Subsidy This value is included in If this fee is not subsidized by merchants, this value is null ( |
CustomerTransaction
This section details the order transactions between the customer and Global-e in the currency used to place the order (the customer transaction currency), as denoted in the CustomerCurrencyCode
property.
Property | Type | Description |
---|---|---|
| Decimal | The total price paid for the order by the customer |
| Decimal | The total price paid by the customer for the products (only) |
| Decimal | The total price of the products after deducting cart and product discounts paid by the customer |
| Decimal | The total shipping price after the discount is paid by the customer. The shipping price, including all shipping fees levied on the customer, such as the |
| Decimal | The duties paid by the customer, including import duties, taxes, and customs clearance fees [CCF]). (Only relevant to international transactions outside the EU). |
| Decimal | The total VAT included in the products or shipping sum paid by the customer (EU to EU transactions), if applicable. |
| Decimal | Shipping VAT rate, if applicable. |
| Decimal | US sales tax paid by the customer (US customers only, as applicable) |
Fees
The following table lists the fees paid by the customer.
Property | Type | Description |
---|---|---|
| Decimal | Customs clearance fee, if not included in the shipping cost |
| Decimal | Cash on delivery fee |
| Decimal | Dangerous goods fee |
| Decimal | Size overcharge fee |
| Decimal | Remote area surcharge fee, if not subsidized by the merchant |
CustomerTransactionsInMerchantCurrency
This section details the order transactions between the customer and Global-e in the currency used to place the order (the customer transaction currency), as denoted in the CustomerCurrencyCode
property.
Global-e converts this amount to merchant base currency, as denoted in the MerchantCurrencyCode
property. Prices are converted by spot exchange rate.
Property | Type | Description |
---|---|---|
| Decimal | The total price paid for the order by the customer |
| Decimal | The total price paid by the customer for the products (only) |
| Decimal | The total price of the products after deducting cart and product discounts paid by the customer |
| Decimal | The total shipping price, after discount, paid by the customer The shipping price includes all shipping fees levied on the customer, such as |
| Decimal | The total VAT sum paid by the customer |
| Decimal | Shipping VAT rate |
| Decimal | The duties paid by the customer. This includes import duties, taxes, and customs clearance fees [CCF]). (Only relevant to international transactions outside the EU). |
| Decimal | US sales tax paid by the customer in Merchant currency |
Fees
The following table details the fees paid by the customer converted to merchant currency.
Property | Type | Description |
---|---|---|
| Decimal | Customer Custom Clearance Fee to be paid by the customer, if not included in the shipping cost |
| Decimal | Cash-on-Delivery fee to be paid by the customer |
| Decimal | Dangerous goods fee to be paid by the customer |
| Decimal | Size overcharge fee to be paid by the customer in the Merchant currency |
| Decimal | Remote area surcharge fee, if not subsidized by the merchant |
Product Information
Property | Type | Description |
---|---|---|
| String | The name of the product 2 Pack Regular Fit Checked Easy Iron Shirts |
| String | URL of the product |
| See Categories. | |
| See Brand | |
| String | Product SKU |
| String | Product Group Code |
| String | Secondary Product Code |
| String | Quantity of units |
| Type | Cart Item ID |
| List of strings | Product coupon code If there are multiple coupons, the list of product coupon codes |
| String | Parent Cart Item ID for product bundles |
| String | Option Cart Item ID |
| See Metadata |
Brand
The following table lists brand names and codes received from the cart information.
Property | Type | Description |
---|---|---|
| String | Product brand code |
| String | Product brand name |
Categories
The list of all category names received.
Note
Product categories must be mapped on the Global-e side or passed by default. Ask Global-e to perform the mapping.
Property | Type | Description |
---|---|---|
| String | Product category code |
| String | Product category name |
Metadata
Metadata consists of product attributes that can vary for the same SKU; for example, customization such as engraving text.
Property | Type | Description |
---|---|---|
| String | Attribute key |
| String | Attribute value |
Product Attributes
Attributes consist of information that does not vary for the same SKU; for example, the color or size (in most cases), in a parent or child catalog structure with variants.
Property | Type | Description |
---|---|---|
| String | Attribute Code |
| String | Attribute Name - Custom product attribute name that is used to describe the personalized product |
| String | Attribute Type Code - Custom product attribute value used to describe the personalized product |
Product Prices
This section details the product prices, discounts, VAT (if applicable), and shipping cost estimations, for the transaction between the Merchant and Global-e in the Merchant base currency. All values except for the shipping values, match the base reports.
Property | Type | Description |
---|---|---|
| Decimal | Product list price (before discounts). This property always denotes the product price in the default Merchant country, regardless of The list price is sent in the Cart API. |
| Decimal | Product sale price, before applying any price modifications. This property always denotes the product price in the default merchant country, regardless of |
| Decimal | The total price of all units |
| Decimal | The VAT rate for the product, if applicable |
| Decimal | The price after discount |
This section details the product prices excluding VAT, discounts, and shipping cost estimations, for the transaction between the Merchant and Global-e in the merchant base currency. All values except for the shipping values, match the reconciliation reports.
Property | Type | Description |
---|---|---|
| Decimal | Product list price (before discounts), before applying any price modifications. This property always denotes the product price in the default Merchant country, regardless of The list price is sent in the Cart API. |
| Decimal | The price of the sale |
| Decimal | Total price of all units |
| Decimal | Discounted price |
In the following customer transactions, all prices are in customer currency, according to the values visible on the checkout page.
Property | Type | Description |
---|---|---|
| Decimal | Product list price |
| Decimal | Product selling price |
| Decimal | Total price of all units |
| Decimal | VAT rate (if applicable) |
| Decimal | The discounted price paid by the customer |
In the following customer transactions, all prices are in merchant currency. Prices are converted by exchange spot rate.
Property | Type | Description |
---|---|---|
| Decimal | Product list price converted to merchant currency |
| Decimal | Product sale price as visible to the customer, after applying country coefficient, FX conversion, rounding rule (if applicable), and |
| Decimal | Total price of all the units included in the order converted to merchant currency |
| Decimal | The reduced price (after discounts) of the product in merchant currency. |
Payment Methods
The means with which the customer made the payment (credit card, PayPal, other),
Global-e recommends using the new and enhanced OrderPaymentMethods
instead of the PaymentMethods
listed below (maintained for backward compatibility).
Property | Type | Description |
---|---|---|
| String | The name of the payment method Example: Standard method: credit card (Visa) Alternative method: PayPal |
| String | Payment Method Code |
| Numeric | Payment Method Type ID associated with the payment method type: 0 = Undefined 1 = Credit Card 2 = Local Alternative 3 = Global Alternative 4 = Coupon 5 = Credit Card Installments |
| String | Payment Method Type Name: Undefined, Credit Card, Local Alternative, Global Alternative, Coupon, Credit Card Instalments |
Discount Information
General Discount Information
Parameter | Type | Description |
---|---|---|
| String | The discount description |
| String | The discount code |
| Integer long | The cart discount type. Discount related to the amount, coupon, or another promotion value. The Discount Type Option Values table describes one of the following possible values of |
| String | Discount type name, such as shipping discount, cart discount, product discount, and more. |
| String | The coupon code |
| String | Cart Item Id. The connection between the discount and product by cartItemId in product - this is null if the discount does not refer to a specific product but was cart level (distributed between products) |
Discount
Property | Type | Description |
---|---|---|
| Decimal | Discount value in the Merchant base currency including the local VAT, before applying any price modifications. This property always denotes the discount value in the default Merchant’s country, regardless of UseCountryVAT for the customer’s current country. |
VATRate | Decimal | The VAT rate applied to this discount (if applicable) |
LocalVATRate | Decimal (optional) | The VAT rate applied to this discount if the order is placed by the customer. This value must be specified if UseCountryVAT for the current Country is TRUE and therefore, the VATRate property actually denotes the VAT for the target country. |
DiscountValue | Decimal | Discount value as displayed to the customer, after applying country coefficient, FX conversion, and IncludeVAT handling |
Name | String | Discount name |
Description | String (optional) | Discount textual description |
CouponCode | String (optional) | Merchant’s coupon code used for this discount (applicable to coupon-based discounts only) |
ProductCartItemId | String (optional) | Identifier of the product cart item related to this discount on the Merchant’s site. This property may be optionally specified in the Cart API only so that the same value could be posted back when creating the order on the Merchant’s site with SendOrderToMerchant API. |
DiscountCode | String (optional) | Discount code used to identify the discount on the Merchant’s site. This property may be optionally specified in the Cart API only so that the same value could be posted back when creating the order on the Merchant’s site with SendOrderToMerchant API. |
LoyaltyVoucherCode | String (optional) | Code used on the Merchant’s site to identify the loyalty voucher on which this discount is based. |
DiscountType | Int64 | The DiscountType Option Value table describes one of the following possible values of DiscountTypeOptions enumeration denoting a type of a discount. |
DiscountType Option Value
DiscountType Value | Name | Description |
---|---|---|
1 | Cart discount | Discount related to volume, amount, coupon, or another promotion value |
2 | Shipping discount | Discount aimed at subsidizing international shipping |
3 | Loyalty points discount | Discount applied against the Merchant’s loyalty points to be spent for this purchase |
4 | Duties discount | Discount aimed at sponsoring taxes & duties pre-paid by the customer in Global-e checkout |
5 | Checkout loyalty points discount | Discount applied against the Merchant’s loyalty points in Global-e checkout |
6 | Payment charge | Discount aimed at subsidizing the “Cash on Delivery” fee |
Parameter | Type | Description |
---|---|---|
| Decimal | Discount Value |
| Integer | The merchant Local VAT rate |
Parameter | Type | Description |
---|---|---|
| Decimal | Discount Value |
Parameter | Type | Description |
---|---|---|
| Decimal | Discount Value |
| Integer | VAT Amount |
Customer Transactions in Merchant Currency
Parameter | Type | Description |
---|---|---|
| Decimal | The price of the customer discount in customer currency |
| Integer | VAT amount |
OrderPaymentMethods
OrderPaymentMethods
provide comprehensive information about the payment methods used by the customer to pay for the order; it includes a breakdown of the amount paid as well as a gift card indication, when applicable.
Method | Type | Description |
---|---|---|
| Array | A list of payment methods used to place the order. |
Each payment method contains the following elements.
Property | Type | Description |
---|---|---|
| Integer | The ID of the payment method in the Global‑e system. |
| String | The name of the payment method. |
| Boolean | Used by the merchant to identify the gift card payment method. |
| String | Key-values pairs from the gift card merchant app setting (in the Global‑e system). Null for regular payments (without gift cards).
|
| Decimal | The amount paid in customer currency. |
| Decimal | The amount paid in merchant currency, using spot conversion. |
| Numeric | Payment Method Type ID associated with the payment method type: 0 = Undefined 1 = Credit Card 2 = Local Alternative 3 = Global Alternative 4 = Coupon 5 = Credit Card Instalments |
| String | Payment Method Type Name: Undefined, Credit Card, Local Alternative, Global Alternative, Coupon, Credit Card Instalments |
"OrderPaymentMethods": [, { "PaymentMethodId": 1, "PaymentMethodName": "Visa", "IsGiftCard": false, "GiftCardFields": "null", "PaidAmountInCustomerCurrency": 388.0, "PaidAmountInMerchantCurrency": 99.0 "PaymentMethodTypeCode": "1" "PaymentMethodTypeName": "Credit Card" }, ] { "PaymentMethodId": 57, "PaymentMethodName": "gift card", "IsGiftCard": true, "GiftCardFields": "[{"FieldApiName":"CardId", "FieldNameResourceId":"checkout.GiftCard.GiftCardId", "FieldValue":"DDB4HB9BDFD466BD","IsId":true}]", "PaidAmountInCustomerCurrency": 388.0, "PaidAmountInMerchantCurrency": 99.0 }, ]
Global‑e Properties
Property | Type | Description |
---|---|---|
| String | URL (Store URL) |
| String | Technical field for Global‑e use; to be disregarded in the merchant implementation. |
| String | Merchant ID, as registered in the Global‑e system |
| String | The title of the transaction |
| String | The name of the server hosting merchant website |
| String | Technical field for Global‑e use; to be disregarded in the merchant implementation. |
| Number | Technical field for Global‑e use; to be disregarded in the merchant implementation. |
| String | Cart token for the cart generated on the Global‑e side by the Cart API method preceding the current cart action. This property is mandatory only for server-side calls. |
| string | The URL of the previous webpage from which a link was followed. |
| Boolean | Receives and logs plain messages and sends them to other logging methods. |
Properties Distribution per Transaction
The following table provides the distribution of properties for each transaction.
ORDER Properties Distribution
Property | Merchant Transactions | Merchant Transactions Excluding Local VAT | Customer Transactions | Customer Transactions in Merchant Currency |
---|---|---|---|---|
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✓ | ✗ | ✗ |
| ✗ | ✓ | ✓ | ✓ |
| ✓ | ✗ | ✓ | ✓ |
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✗ | ✓ | ✓ |
| ✓ | ✓ | ✓ | ✓ |
| ✗ | ✗ | ✓ | ✓ |
| ✗ | ✗ | ✓ | ✓ |
| ✗ | ✗ | ✓ | ✓ |
| ✗ | ✗ | ✓ | ✓ |
PRODUCT Properties Distribution
Property | Merchant Transactions | Merchant Transactions Excluding Local VAT | Customer Transactions | Customer Transactions in Merchant Currency |
---|---|---|---|---|
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✓ | ✓ | ✓ |
DISCOUNTS Properties Distribution
Property | Merchant Transactions | Merchant Transactions Excluding Local VAT | Customer Transactions | Customer Transactions in Merchant Currency |
---|---|---|---|---|
| ✓ | ✓ | ✓ | ✓ |
| ✓ | ✗ | ✓ | ✓ |
Examples
This section provides implementation examples.
Checkout LOAD Step
Key points:
The Checkout Load step is called when the customer visits the checkout page and when switching the country.
The information included in the Checkout Load Step is a subset of the Confirmation step.
{ "CustomerCurrencyCode": "ILS", "MerchantCurrencyCode": "GBP", "IsEU": false, "CustomerDetails":{ "MerchantUserId": "null", } "HubCountryCode": "GB", "ProductInformation": [ { "ProductName": "Chiara Neck Pleat Dress", "ProductURL": "http://johndoe.bglobale.de/dress-8070.html", "Categories": [ { "Code": "20", "Name": "Dresses" } ], "Brand": null, "SKU": "AE117botanical22", "ProductGroupCode": "AE117botanical", "SecondaryProductCode": null, "Quantity": "1", "CartItemId": "308588", "ProductCouponCodes": null, "ParentCartItemID": null, "CartItemOptionId": null, "MetaData": null, "ProductAttributes": [ { "AttributeCode": "color", "AttributeName": "Grey", "AttributeTypeCode": "color" }, { "AttributeCode": "size", "AttributeName": "L", "AttributeTypeCode": "size" } ] "ProductPrices": { "MerchantTransaction": { "ListPrice": 199, "SalePrice": 199.14, "TotalPrice": 199.14, "VATRate": 20, "DiscountedPrice": 199.14 }, "MerchantTransactionExcludingLocalVAT": { "ListPriceExcludingVAT": 165.83, "SalePriceExcludingVAT": 165.95, "TotalPriceExcludingVAT": 165.95, "DiscountedPriceExcludingVAT": 165.95 }, "CustomerTransaction": { "CustomerListPrice": 221, "CustomerSalePrice": 221, "CustomerTotalPrice": 221, "CustomerVATRate": 16, "CustomerDiscountedPrice": 221 }, "CustomerTransactionInMerchantCurrency": { "CustomerListPriceInMerchantCurrency": 200.93, "CustomerSalePriceInMerchantCurrency": 200.93, "CustomerTotalPriceInMerchantCurrency": 200.93, "CustomerDiscountedPriceInMerchantCurrency": 200.93 } } } ], "Discounts": [ { "Description": "Shipping discount provided from fixed price range 145943, negative discount provided to the merchant", "DiscountCode": null, "DiscountTypeId": 2, "DiscountTypeName": "Shipping discount", "CouponCode": "", "ProductCartItemId": null, "DiscountPrices": { "MerchantTransaction": { "Price": -3.67, "VATRate": 20 }, "MerchantTransactionExcludingLocalVAT": { "PriceExcludingVAT": -3.06 }, "CustomerTransaction": { "CustomerPrice": -4.212, "CustomerVATRate": 16 }, "CustomerTransactionInMerchantCurrency": { "CustomerPriceInMerchantCurrency": -3.83, "CustomerVATRate": 16 } } } ], "cultureCode": "en-GB", "InitialCheckoutCultureCode": "en-GB", "URL": null, "CustomURL": null, "MerchantID": 101, "Title": "Global‑e Checkout Load Information", "CustomHostName": null, "PageType": 0, "CartToken": "5b29b332-a798-4682-b803-97759753c8d5", "UrlReferer": null "Dolog": false }
Analytics Implementation and Mapping to Analytics
This section details some basic methods you can use to include the Global-e analytics subscription in your code.
Load the following as a dedicated logic directly in DOM as JavaScript content: subscription, complete data layer mapping, and event execution.
Example: Frontend DOM
<script> var glegem = glegem || function () { (window["glegem"].q = window["glegem"].q || []).push(arguments) }; glegem("OnCheckoutStepLoaded", function (data) { switch (data.StepId) { case data.Steps.LOADED: //fire checkout loaded event break; case data.Steps.CONFIRMATION: if (data.IsSuccess) { gtag("event", "purchase", { transaction_id: data.OrderId, affiliation: "Google Merchandise Store", value: data.details.OrderPrices.MerchantTransaction.EstimatedTotalPrice, tax: data.details.OrderPrices.MerchantTransaction.TotalVAT, shipping: data.details.orderPrices.MerchantTransaction.EstimatedShippingCost, currency: data.details.MerchantCurrencyCode, items: { { item_id: data.details.ProductInformation[0].SKU, item_name: data.details.ProductInformation[0].ProductName, affiliation: "Google Merchandise Store", currency: "USD", index: 0, location_id: "ChIJIQBpAG2ahYAR_6128GcTUEo", price: data.details.ProductInformation[0].ProductPrices .CustomerTransactionInMerchantCurrency .CustomerDiscountedPriceInMerchantCurrency, quantity: data.details.ProductInformation[0].Quantity } ] }); } break; } }); </script>
Use a tag manager such as GTM to load the subscription, data mapping, and events, as a dedicated logic.
You can also perform this as inline data mapping and tag-based triggers, depending on current practices.
Example: GTM Tag Manager
<script> var glegem = window["glegem"] || function() { (window["glegem"].q = window["glegem"].q || []).push(arguments); }; glegem("OnCheckoutStepLoaded", function(data) { switch(data.StepId) { case data.Steps.LOADED: break; case data.Steps.CONFIRMATION: console.log(data); if(data.IsSuccess) { if(typeof dataLayer !== "undefined") { let products = new Array(); for(let i = 0; i < data.details.ProductInformation.length; i++) { let tempProducts = {}; tempProducts.id = data.details.ProductInformation[i].SKU; tempProducts.name = data.details.ProductInformation[i].ProductName; tempProducts.price = data.details.ProductInformation[i].ProductPrices.MerchantTransaction.SalePrice; tempProducts.quantity = data.details.ProductInformation[i].Quantity; products.push(tempProducts); } var couponCode = ''; var discountAmount = ''; for(let i = 0; i < data.details.Discounts.length; i++) { if(data.details.Discounts[i].CouponCode != '' && data.details.Discounts[i].DiscountTypeId == 1) { couponCode = data.details.Discounts[i].CouponCode; discountAmount = data.details.Discounts[i].DiscountPrices.MerchantTransaction.Price; } } dataLayer.push({ 'event': 'purchase_GE', 'ecommerce': { 'purchase': { 'actionField': { 'id': data.OrderId, 'revenue': data.details.OrderPrices.MerchantTransaction.TotalProductsPrice, 'coupon': couponCode, 'discountAmount': discountAmount }, 'products': products }, "currencyCode": data.details.MerchantCurrencyCode, } }); } } break; } }); </script>
GB Orders
GB Order Sent to the US with Sales Tax and Duties and Taxes Paid Separately at Checkout (Optional DDP)
Key points :
The VAT is applied on the Merchant transaction between Global-e and the brand.
Duties paid as part of the product price are featured as such and described under a subsidy discount object.
The applicable US sales tax is featured in a dedicated field.
US Orders
US Order Sent to any Country as Optional DDP
Key points:
There is no local tax applicable to the transaction between Global-e and the brand,
Duties & taxes paid separately by the customer on the checkout page are featured as a transaction amount for the customer transaction but not between Global-e and the brand.
There is no VAT applicable to the customer or the brand (local taxes are handled under Duties & Taxes).
{ "OrderID": "GE3593948US", "ReplacementOrder": false, "CustomerCurrencyCode": "EUR", "MerchantCurrencyCode": "USD", "IsEU": false, "CustomerDetails": { "MerchantUserId": "VCustomer123", "ShippingAddress": { "ShippingFirstName": "Valued", "ShippingLastName": "Customer", "ShippingAddress1": "Basel 25", "ShippingAddress2": "", "ShippingCity": "Petah tikva", "ShippingCountryName": null, "ShippingCountryCode": null, "ShippingState": null, "ShippingStateCode": null, "ShippingCompany": "Global‑e", "ShippingZipCode": "49510", "ShippingPhoneNumber": "0732041382" }, "BillingAddress": { "BillingFirstName": "Valued", "BillingLastName": "Customer", "BillingAddress1": "Basel 25", "BillingAddress2": "", "BillingCity": "Petah tikva", "BillingCountryName": null, "BillingCountryCode": null, "BillingState": null, "BillingStateCode": null, "BillingCompany": "Global‑e", "BillingZipCode": "49510", "BillingPhoneNumber": "0732041382", "BillingEmail": "info@global-e.com" } }, "HubCountryCode": "US", "Region": null, "SiteURL": "https://dev05-store-modcloth.demandware.net/s/modcloth/home", "DutiesGuaranteed": true, "ShippingMethodName": "RRDonnelley DDP", "ShippingMethodType": "Tracked Post", "DeliveryDaysRangeFrom": 6, "DeliveryDaysRangeTo": 10, "OrderPrices": { "MerchantTransaction": { "EstimatedTotalPrice": 51.14, "TotalProductsPrice": 56.18, "TotalDiscountedProductsPrice": 56.18, "EstimatedShippingCost": 5.04, "ShippingVATRate": 0, "DutiesAndTaxes": 0, "ProductTotalVAT": 0, "TotalVAT": 0, "RemoteAreaSurchargeFee": 0 }, "MerchantTransactionExcludingLocalVAT": { "EstimatedTotalPriceExcludingVAT": 51.14, "TotalProductsPriceExcludingVAT": 56.18, "TotalDiscountedProductsPriceExcludingVAT": 56.18, "EstimatedShippingCostExcludingVAT": 5.04, "CustomerShippingPriceExcludingVAT": 5.55, "DutiesAndTaxesExcludingVAT": 0, "RemoteAreaSurchargeFeeExcludingVAT": 0 }, "CustomerTransaction": { "CustomerTotalPrice": 69.06, "CustomerTotalProductsPrice": 52, "CustomerTotalDiscountedProductsPrice": 52, "CustomerShippingPrice": 5.55, "CustomerDutiesAndTaxes": 11.51, "CustomerTotalVAT": 0, "CustomerShippingVATRate": 0, "CustomerUSSalesTax": 0, "Fees": { "CustomerCCF": 0, "CustomerCODFee": 0, "CustomerDangerousGoodsFee": 0, "CustomerSizeOverchargeFee": 0, "CustomerRemoteAreaSurchargeFee": 0 } }, "CustomerTransactionInMerchantCurrency": { "CustomerTotalPriceInMerchantCurrency": 81.26, "CustomerTotalProductsPriceInMerchantCurrency": 61.18, "CustomerTotalDiscountedProductsPriceInMerchantCurrency": 61.18, "CustomerShippingPriceInMerchantCurrency": 6.53, "CustomerVATInMerchantCurrency": 0, "CustomerShippingVATRate": 0, "CustomerDutiesAndTaxesInMerchantCurrency": 13.54, "CustomerUSSalesTaxInMerchantCurrency": 0, "Fees": { "CustomerCCFInMerchantCurrency": 0, "CustomerCODFeeInMerchantCurrency": 0, "CustomerDangerousGoodsFeeInMerchantCurrency": 0, "CustomerSizeOverchargeFeeInMerchantCurrency": 0, "CustomerRemoteAreaSurchargeFeeInMerchantCurrency": 0 } } }, "ProductInformation": [ { "ProductName": "In Knit to Win It Cotton Cardigan in L/XL", "ProductURL": "shop/tops/modcloth-in-knit-to-win-it-cotton-cardigan-in-ivory", "Categories": null, "Brand": null, "SKU": "100000370403", "ProductGroupCode": "10126810", "SecondaryProductCode": null, "Quantity": "1", "CartItemId": "1", "ProductCouponCodes": null, "ParentCartItemID": null, "CartItemOptionId": null, "MetaData": null, "ProductAttributes": [ { "AttributeCode": "WHT", "AttributeName": "White", "AttributeTypeCode": "colour" }, { "AttributeCode": "L/XL", "AttributeName": "L/XL", "AttributeTypeCode": "size" }, { "AttributeCode": "ModCloth", "AttributeName": "ModCloth", "AttributeTypeCode": "brand" } ], "ProductPrices": { "MerchantTransaction": { "ListPrice": 56.18, "SalePrice": 56.18, "TotalPrice": 56.18, "VATRate": 0, "DiscountedPrice": 56.18 }, "MerchantTransactionExcludingLocalVAT": { "ListPriceExcludingVAT": 56.18, "SalePriceExcludingVAT": 56.18, "TotalPriceExcludingVAT": 56.18, "DiscountedPriceExcludingVAT": 56.18 }, "CustomerTransaction": { "CustomerListPrice": 52, "CustomerSalePrice": 52, "CustomerTotalPrice": 52, "CustomerVATRate": 0, "CustomerDiscountedPrice": 52 }, "CustomerTransactionInMerchantCurrency": { "CustomerListPriceInMerchantCurrency": 61.18, "CustomerSalePriceInMerchantCurrency": 61.18, "CustomerTotalPriceInMerchantCurrency": 61.18, "CustomerDiscountedPriceInMerchantCurrency": 61.18 } } } ], "PaymentMethods": [ { "PaymentMethodName": "Paypal", "PaymentMethodCode": "4", "PaymentMethodTypeCode": "3", "PaymentMethodTypeName": "Global Alternative" } ], "Discounts": [ { "Description": "Shipping discount provided from fixed price range 256683", "DiscountCode": null, "DiscountTypeId": 2, "DiscountTypeName": "Shipping discount", "CouponCode": "", "ProductCartItemId": null, "DiscountPrices": { "MerchantTransaction": { "Price": 5.04, "VATRate": 0 }, "MerchantTransactionExcludingLocalVAT": { "PriceExcludingVAT": 5.04 }, "CustomerTransaction": { "CustomerPrice": 4.67, "CustomerVATRate": 0 }, "CustomerTransactionInMerchantCurrency": { "CustomerPriceInMerchantCurrency": 5.49, "CustomerVATRate": 0 } } } ], "OrderPaymentMethods": [ { "PaymentMethodId": 4, "PaymentMethodName": "Paypal", "IsGiftCard": false, "GiftCardFields": "null", "PaidAmountInCustomerCurrency": 461.25, "PaidAmountInMerchantCurrency": 97.31578659366438, "PaymentMethodTypeCode": "3", "PaymentMethodTypeName": "Global Alternative" } ] "cultureCode": "en-GB", "InitialCheckoutCultureCode": "en-GB", "URL": null, "CustomURL": null, "CheckoutStep": null, "MerchantID": 506, "Title": "Global‑e Order Transaction", "HostName": "https://www2.bglobale.com/", "CustomHostName": null, "PageType": 0, "CartToken": "2cea3b52-08aa-46dd-9d0f-5a663305149c", "CountryCode": "AT", "UrlReferer": null, "DoLog": false }
EU Order with Customer VAT and Merchant VAT
Key points:
Merchant and Customer VAT rates can differ if they are applied:
between Global-e and the brand - or -
between Global-e and the customer (EU distance selling VAT rules)
As a result, VAT inclusive product prices also differ.
No duties applicable
{ "OrderID": "GE103831808GB", "ReplacementOrder": false, "CustomerCurrencyCode": "EUR", "MerchantCurrencyCode": "GBP", "IsEU": true, "CustomerDetails": { "MerchantUserId": "IvanT123", "ShippingAddress": { "ShippingFirstName": "Ivan", "ShippingLastName": "Test", "ShippingAddress1": "Germany, Rontgenstr. 9", "ShippingAddress2": null, "ShippingCity": "Maxdorf", "ShippingCountryName": "Germany", "ShippingCountryCode": "DE", "ShippingState": null, "ShippingStateCode": null, "ShippingCompany": "Israel", "ShippingZipCode": "6713333", "ShippingPhoneNumber": "0000000000" }, "BillingAddress": { "BillingFirstName": "Ivan", "BillingLastName": "Test", "BillingAddress1": "Germany, Rontgenstr. 9", "BillingAddress2": null, "BillingCity": "Maxdorf", "BillingCountryName": "Germany", "BillingCountryCode": "DE", "BillingState": null, "BillingStateCode": null, "BillingCompany": "Israel", "BillingZipCode": "6713333", "BillingPhoneNumber": "0000000000", "BillingEmail": "ivan.koshcheiev@global-e.com" } }, "HubCountryCode": "GB", "Region": null, "SiteURL": "http://anna.bglobale.de/", "DutiesGuaranteed": false, "ShippingMethodName": "DHL Road", "ShippingMethodType": "Standard Courier", "DeliveryDaysRangeFrom": 4, "DeliveryDaysRangeTo": 5, "OrderPrices": { "MerchantTransaction": { "EstimatedTotalPrice": 202.81, "TotalProductsPrice": 199.14, "TotalDiscountedProductsPrice": 199.14, "EstimatedShippingCost": -3.67, "ShippingVATRate": 20, "DutiesAndTaxes": 0, "ProductTotalVAT": 33.19, "TotalVAT": 33.8, "RemoteAreaSurchargeFee": 0 }, "MerchantTransactionExcludingLocalVAT": { "EstimatedTotalPriceExcludingVAT": 169.01, "TotalProductsPriceExcludingVAT": 165.95, "TotalDiscountedProductsPriceExcludingVAT": 165.95, "EstimatedShippingCostExcludingVAT": -3.06, "CustomerShippingPriceExcludingVAT": 12.93, "DutiesAndTaxesExcludingVAT": 0, "RemoteAreaSurchargeFeeExcludingVAT": 0 }, "CustomerTransaction": { "CustomerTotalPrice": 236, "CustomerTotalProductsPrice": 221, "CustomerTotalDiscountedProductsPrice": 221, "CustomerShippingPrice": 15, "CustomerDutiesAndTaxes": 0, "CustomerTotalVAT": 32.55, "CustomerShippingVATRate": 16, "CustomerUSSalesTax": 0, "Fees": { "CustomerCCF": 0, "CustomerCODFee": 0, "CustomerDangerousGoodsFee": 0, "CustomerSizeOverchargeFee": 0, "CustomerRemoteAreaSurchargeFee": 0 } }, "CustomerTransactionInMerchantCurrency": { "CustomerTotalPriceInMerchantCurrency": 214.57, "CustomerTotalProductsPriceInMerchantCurrency": 200.93, "CustomerTotalDiscountedProductsPriceInMerchantCurrency": 200.93, "CustomerShippingPriceInMerchantCurrency": 13.64, "CustomerVATInMerchantCurrency": 29.59, "CustomerShippingVATRate": 16, "CustomerDutiesAndTaxesInMerchantCurrency": 0, "CustomerUSSalesTaxInMerchantCurrency": 0, "Fees": { "CustomerCCFInMerchantCurrency": 0, "CustomerCODFeeInMerchantCurrency": 0, "CustomerDangerousGoodsFeeInMerchantCurrency": 0, "CustomerSizeOverchargeFeeInMerchantCurrency": 0, "CustomerRemoteAreaSurchargeFeeInMerchantCurrency": 0 } } }, "ProductInformation": [ { "ProductName": "Chiara Neck Pleat Dress", "ProductURL": "http://johndoe.bglobale.de/chiara-neck-pleat-dress-8070.html", "Categories": [ { "Code": "20", "Name": "Dresses" } ], "Brand": null, "SKU": "AE117botanical22", "ProductGroupCode": "AE117botanical", "SecondaryProductCode": null, "Quantity": "1", "CartItemId": "308588", "ProductCouponCodes": null, "ParentCartItemID": null, "CartItemOptionId": null, "MetaData": null, "ProductAttributes": null, "ProductPrices": { "MerchantTransaction": { "ListPrice": 199, "SalePrice": 199.14, "TotalPrice": 199.14, "VATRate": 20, "DiscountedPrice": 199.14 }, "MerchantTransactionExcludingLocalVAT": { "ListPriceExcludingVAT": 165.83, "SalePriceExcludingVAT": 165.95, "TotalPriceExcludingVAT": 165.95, "DiscountedPriceExcludingVAT": 165.95 }, "CustomerTransaction": { "CustomerListPrice": 221, "CustomerSalePrice": 221, "CustomerTotalPrice": 221, "CustomerVATRate": 16, "CustomerDiscountedPrice": 221 }, "CustomerTransactionInMerchantCurrency": { "CustomerListPriceInMerchantCurrency": 200.93, "CustomerSalePriceInMerchantCurrency": 200.93, "CustomerTotalPriceInMerchantCurrency": 200.93, "CustomerDiscountedPriceInMerchantCurrency": 200.93 } } } ], "PaymentMethods": [ { "PaymentMethodName": "Visa", "PaymentMethodCode": "1", "PaymentMethodTypeCode": "1", "PaymentMethodTypeName": "Credit Card" } ], "Discounts": [ { "Description": "Shipping discount provided from fixed price range 145943, negative discount provided to the merchant", "DiscountCode": null, "DiscountTypeId": 2, "DiscountTypeName": "Shipping discount", "CouponCode": "", "ProductCartItemId": null, "DiscountPrices": { "MerchantTransaction": { "Price": -3.67, "VATRate": 20 }, "MerchantTransactionExcludingLocalVAT": { "PriceExcludingVAT": -3.06 }, "CustomerTransaction": { "CustomerPrice": -4.212, "CustomerVATRate": 16 }, "CustomerTransactionInMerchantCurrency": { "CustomerPriceInMerchantCurrency": -3.83, "CustomerVATRate": 16 } } } ], "OrderPaymentMethods": [ { "PaymentMethodId": 1, "PaymentMethodName": "Visa", "IsGiftCard": false, "GiftCardFields": "null", "PaidAmountInCustomerCurrency": 461.25, "PaidAmountInMerchantCurrency": 97.31578659366438, "PaymentMethodTypeCode": "1", "PaymentMethodTypeName": "Credit Card" } ], "cultureCode": "en-GB", "InitialCheckoutCultureCode": "en-GB", "URL": null, "CustomURL": null, "CheckoutStep": null, "MerchantID": 101, "Title": "Global‑e Order Transaction", "HostName": "https://qa.bglobale.com/", "CustomHostName": null, "PageType": 0, "CartToken": "5b29b332-a798-4682-b803-97759753c8d5", "CountryCode": "DE", "UrlReferer": null }
Advanced Analytics
Advanced Analytics (Client Events)
The Global‑e checkout provides advanced client behavior data collection.
When specific actions are performed by the customer during the checkout process, Global-e triggers events to enable you to track your account activity and returns the relevant data in JSON format. You can map this data to your existing analytics implementation or tracking solution so that you can create conversion reports in your analytics dashboard.
Client Events
The following table describes the client events that are currently available. The checkout analytics event triggers these events.
Event Name | Event Data | Description |
---|---|---|
INPUT_BLUR | id - element id name - element name value - element value | This event is triggered when a customer leaves a text input field |
CHECKBOX_CHANGE | id - sid name - checkbox name value - element value checked - checkbox status ( | This event is triggered when a customer clicks a checkbox |
BUTTON_CLICKED | id - Button ID. Example: " tag - element tag name. Example: " name - element name when available text - button text. Example: " action - button action description when available. Example: " button additional data success - Indicates if payment went through or if the coupon code is successful error - If the payment is not accepted (meaning success property is false), the error field details the payment failure reasons. Possible errors for Payment:
Possible errors for coupon codes:
errorFields - The checkout form field that caused the validation error. If there are multiple validation errors, they are indicated in this field separated by a comma. See Examples of Click Events further below. | This event is triggered when a client clicks the button |
STORE_SELECTION | No data | This event is triggered when a client clicks the ship to shop option (when applicable) |
SHIPPINGMETHOD_SELECTION | id - shipping method ID price - shipping method price | This event is triggered when a client selects a shipping method |
TAX_OPTION_SELECTED | id - selected tax option possible values: | This event is triggered when a client selects a tax option |
PAYMENTMETHOD_CHANGED | id - payment method ID name - payment method name (e.g. Visa, MasterCard, etc.) | This event is triggered when a client selects a payment method |
COMBO_CHANGED | id - element ID name - element name value - selected value | This event is triggered when a client changes a value in a select list |
ADD_NEW_SHIPPING_ADDRESS | No data | This event is triggered when a client selects to add a new shipping address |
ON_PAGE_LOAD | id - page identification, possible values: | This event is triggered when the Checkout page loads |
ON_CLIENT_ERROR | message - error message url - error URL lineNumber - error line number | This event is triggered when a client-side error occurs |
ON_SERVER_ERROR | process - the checkout process that fails error - actual error message | This event is triggered when a server-side error occurs |
CheckoutFailure | Returns failureCode with one of the following values:
| This event is triggered when there is a failure to place an order during checkout |
BILLING_ADDRESS_COMPLETED | No additional data is required | This event is triggered when all mandatory billing address fields have been filled and verified (a green checkmark is displayed next to each field to confirm verification). |
SHIPPING_ADDRESS_COMPLETED | SHIPPING_ADDRESS_COMPLETED - Default The shipping address is the same as the billing address (default):
| This event is triggered when the shipping and billing addresses are the same (default) and all mandatory fields in the billing address have been filled successfully (a green checkmark is displayed next to each field to confirm verification). |
SHIPPING_ADDRESS_COMPLETED - Collection Point The shipping address is a collection point.
| This event is triggered when the customer selects Collection Point as the shipping address and confirms the selected collection point in the popup (select collection point popup). | |
SHIPPING_ADDRESS_COMPLETED - Alternative The shipping address and billing address are different.
| This event is triggered when the customer selects Alternative as the shipping address and all mandatory fields in the shipping address have been filled successfully (a green checkmark is displayed next to each field to confirm verification). | |
SHIPPING_ADDRESS_COMPLETED - Store The shipping address is a store.
| This event is triggered when the customer selects Store as the shipping address and confirms the selected store in the popup (select store popup). |
Examples of Click Events
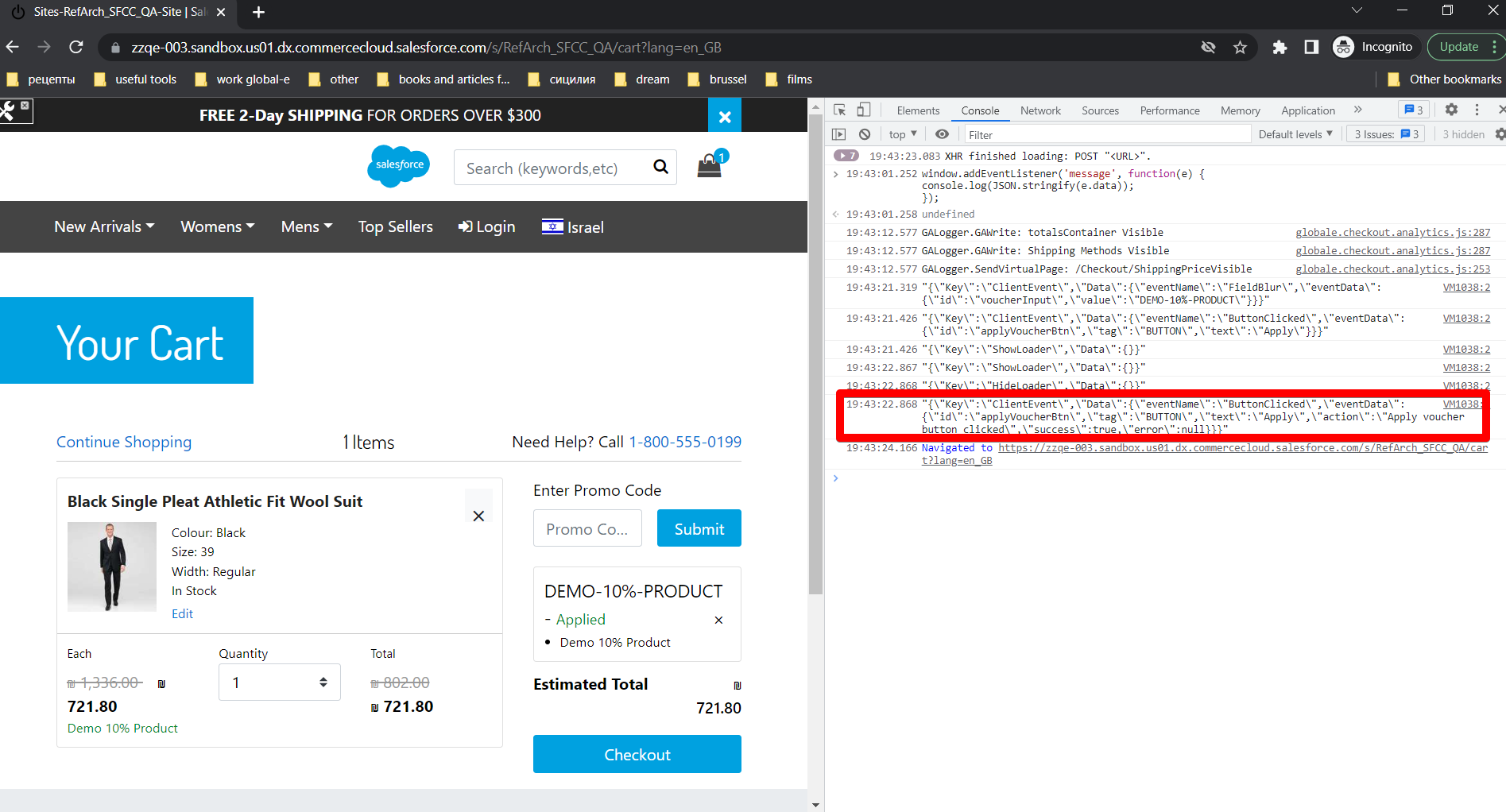
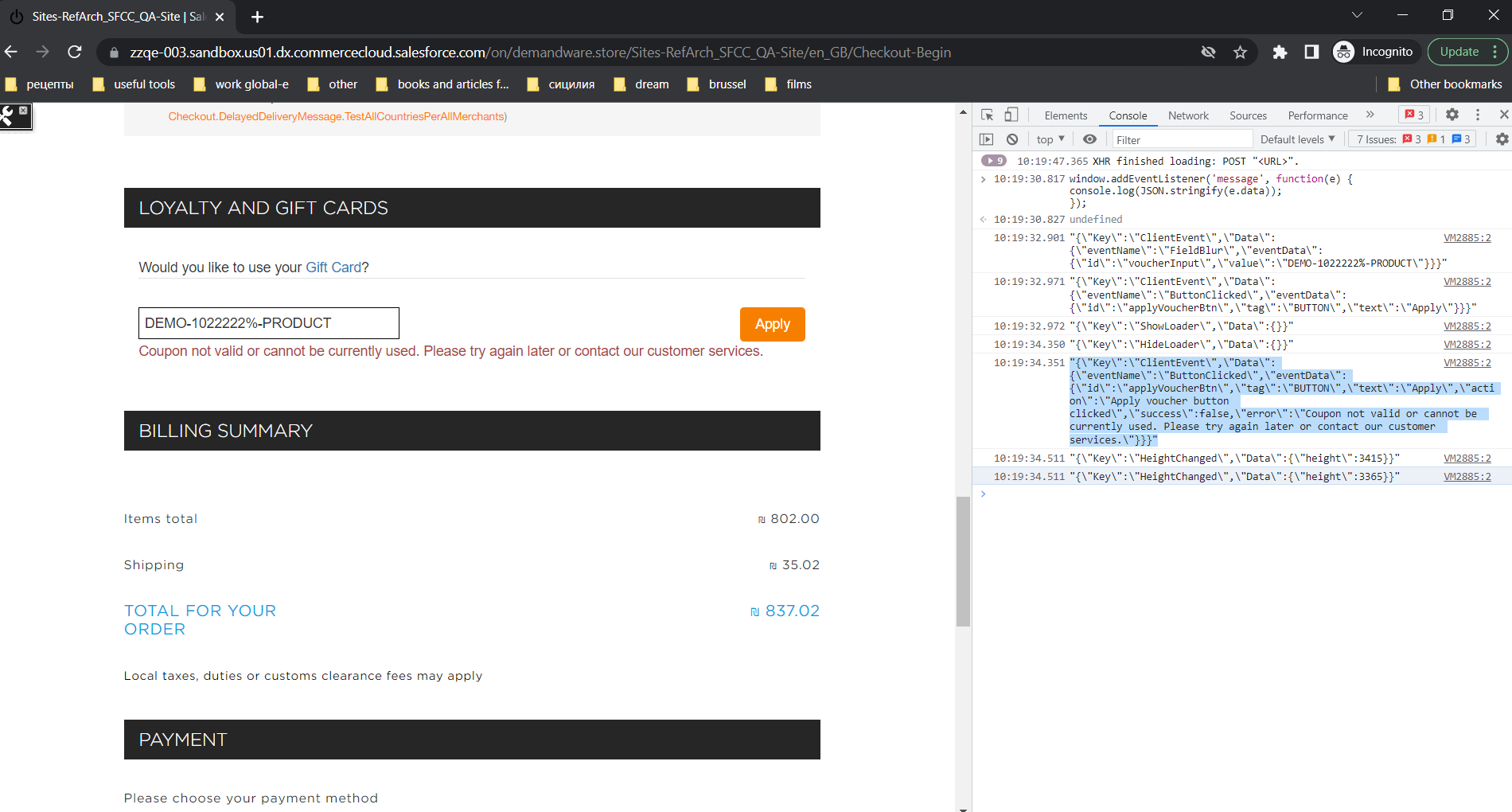
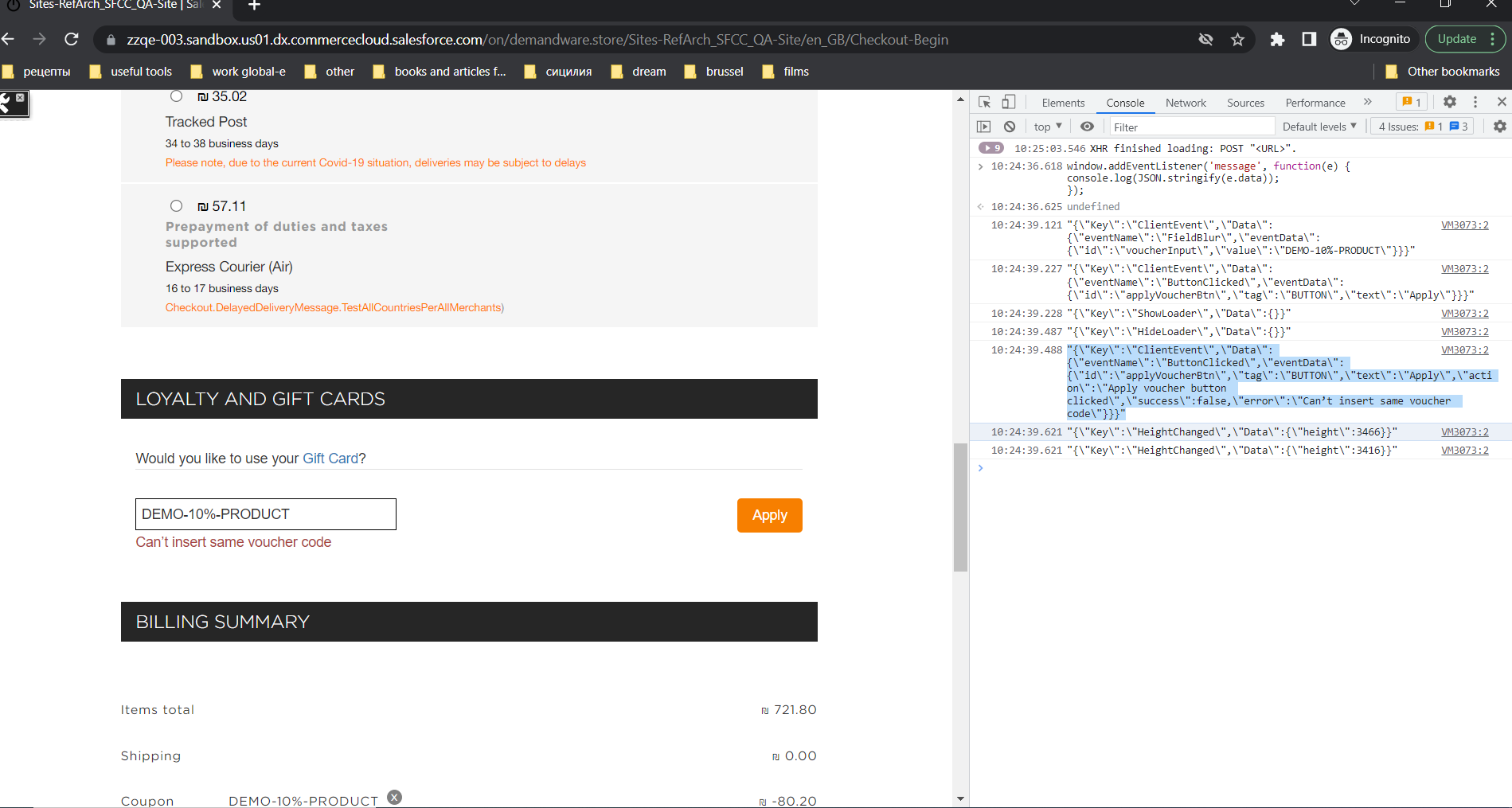
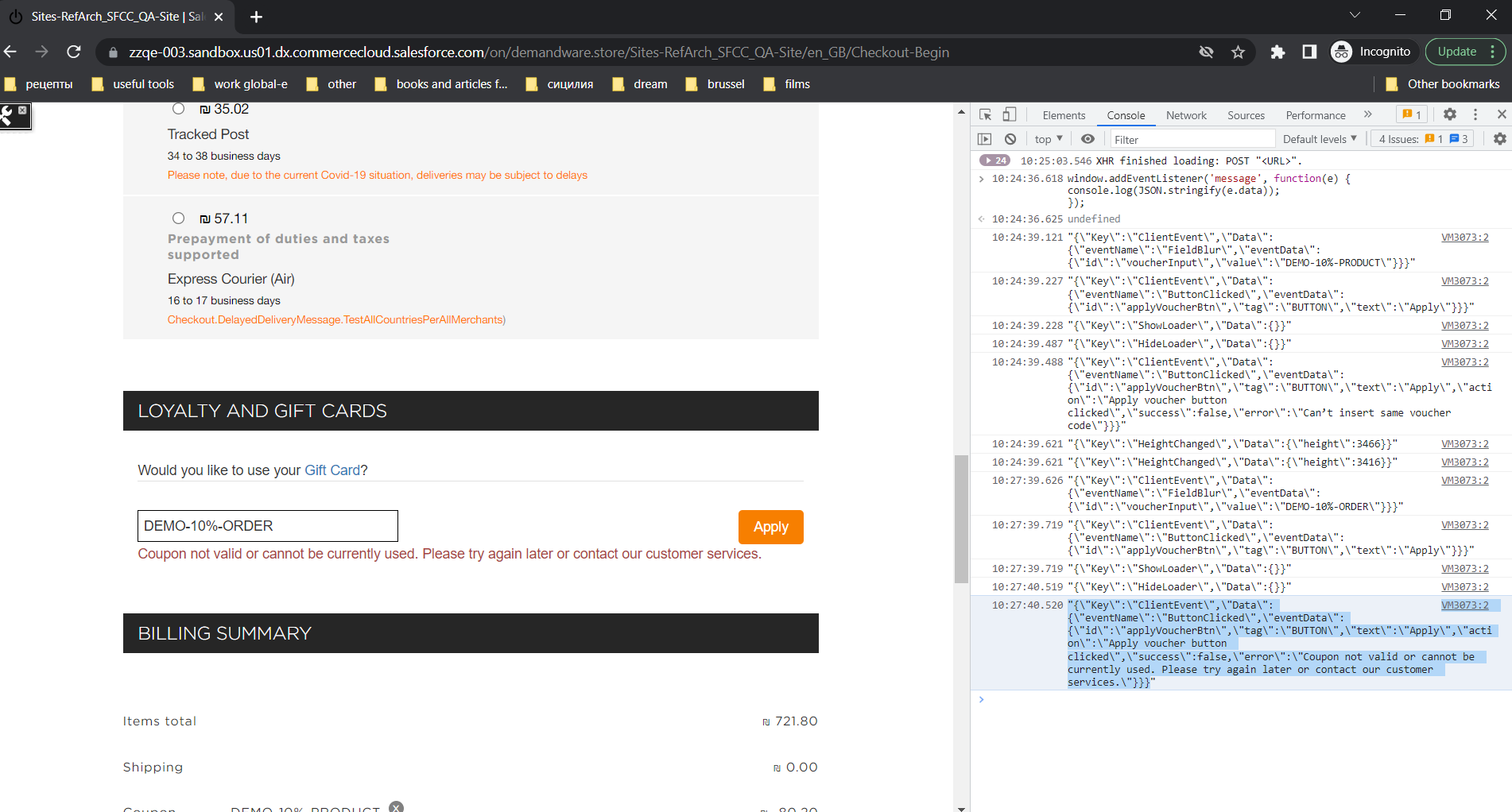
Examples of Shipping and Billing Events
This example shows that both the billing and shipping addresses are the same and that all mandatory fields have been completed successfully. This is the default.
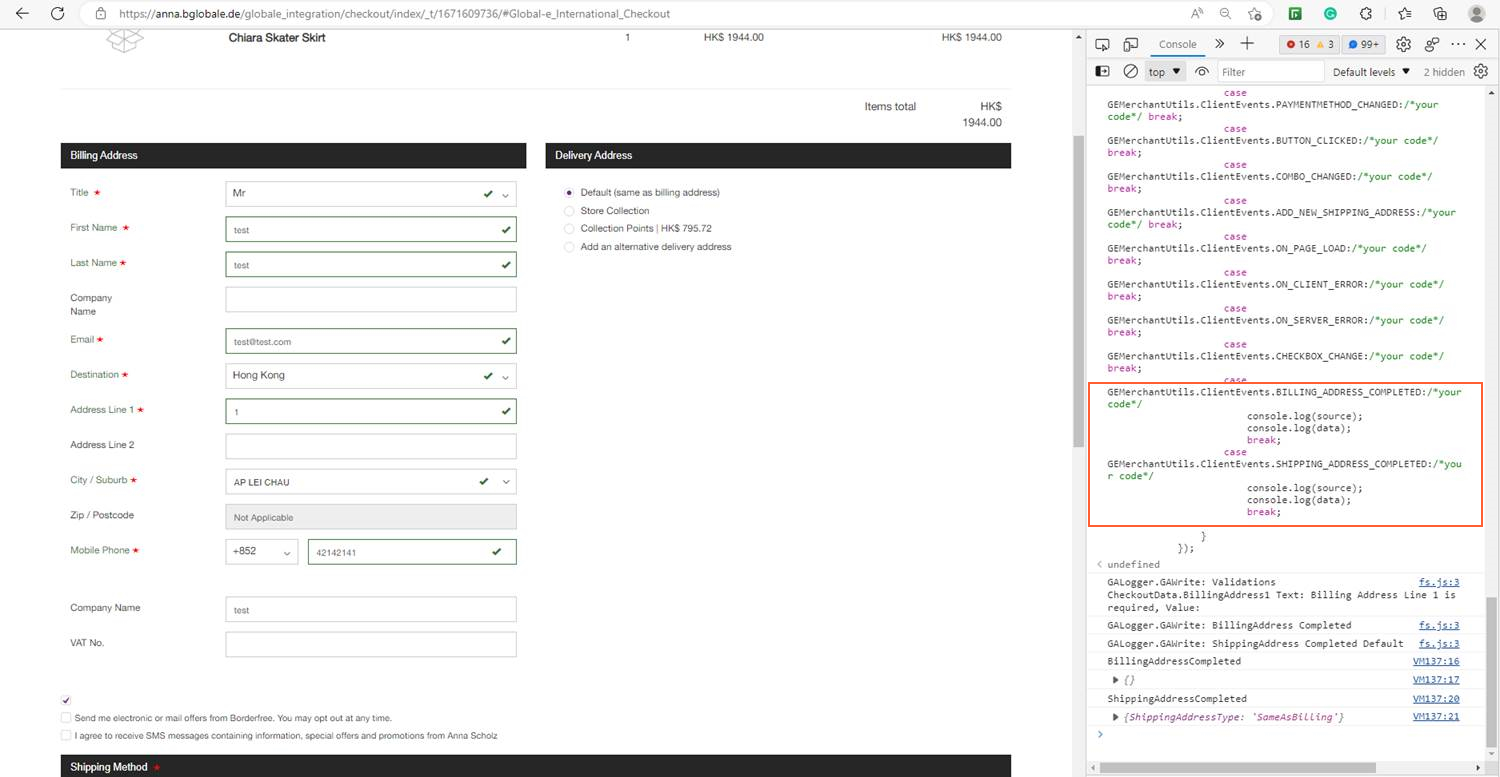
This example shows that the customer has selected a Collection Point for the Shipping Address and that all mandatory fields have been completed successfully.
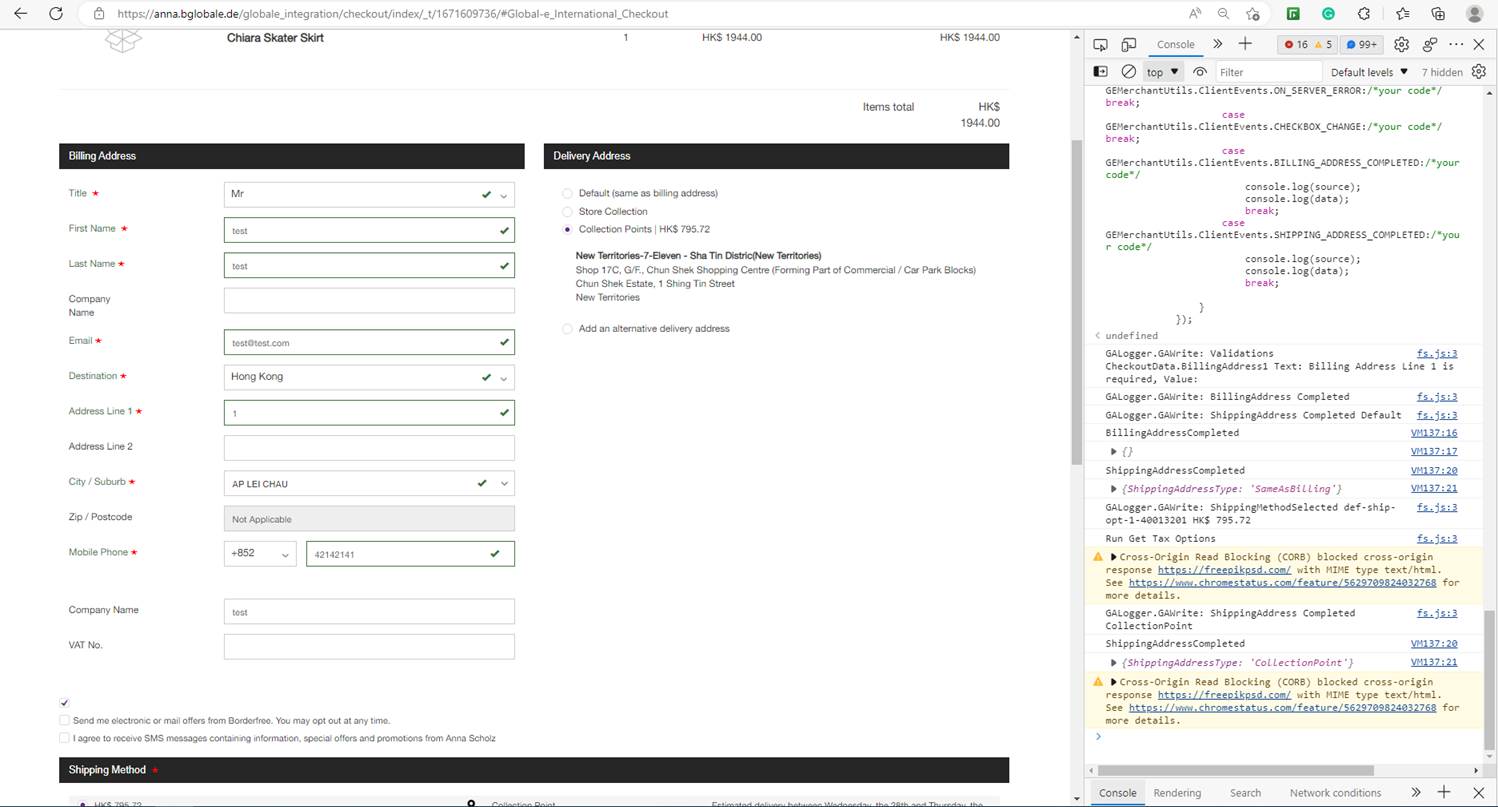
This example shows that the customer has selected a different shipping address (alternative) than that of the billing address and that all mandatory fields have been completed successfully.
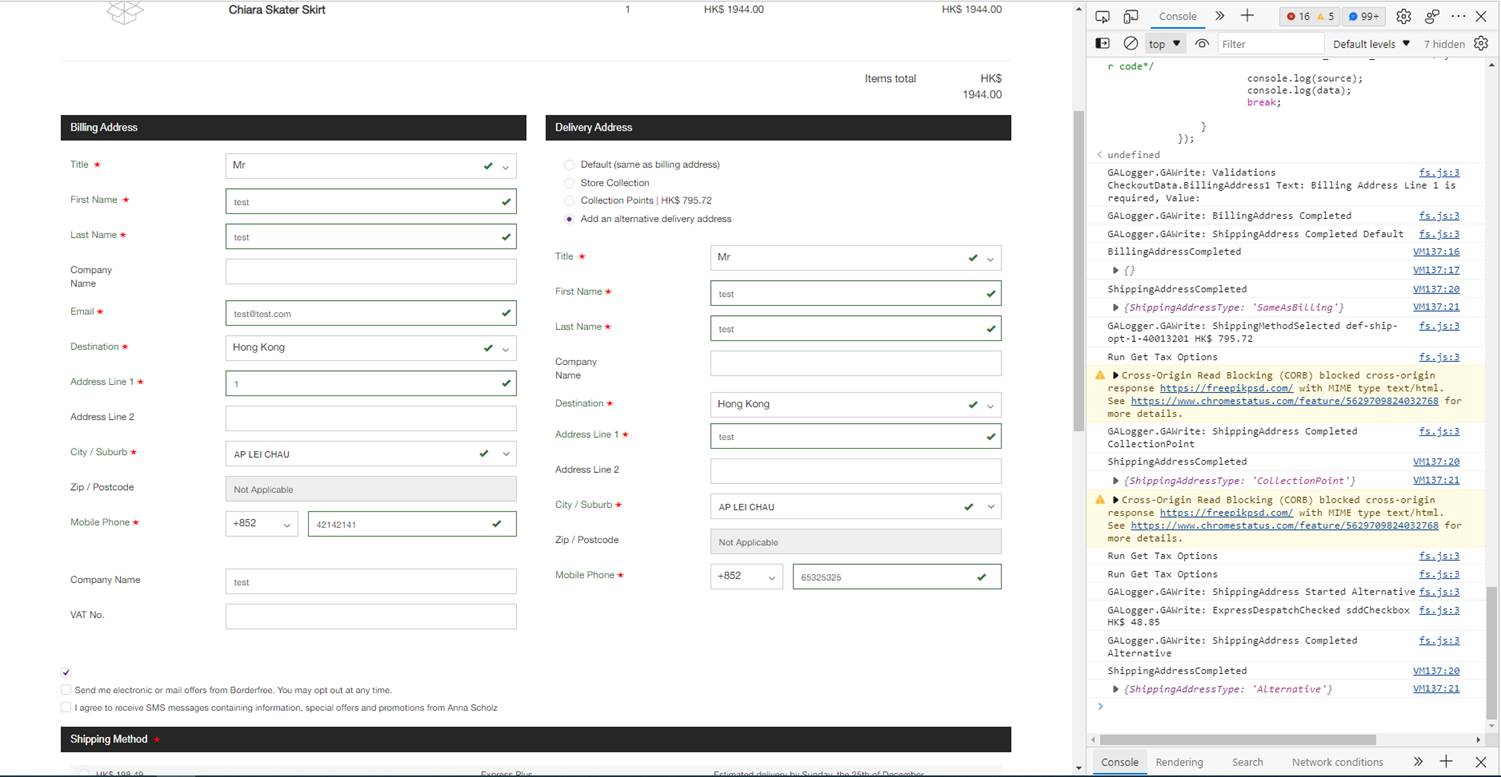
This example shows that the customer has selected a store for the shipping address and that all mandatory fields have been completed successfully.
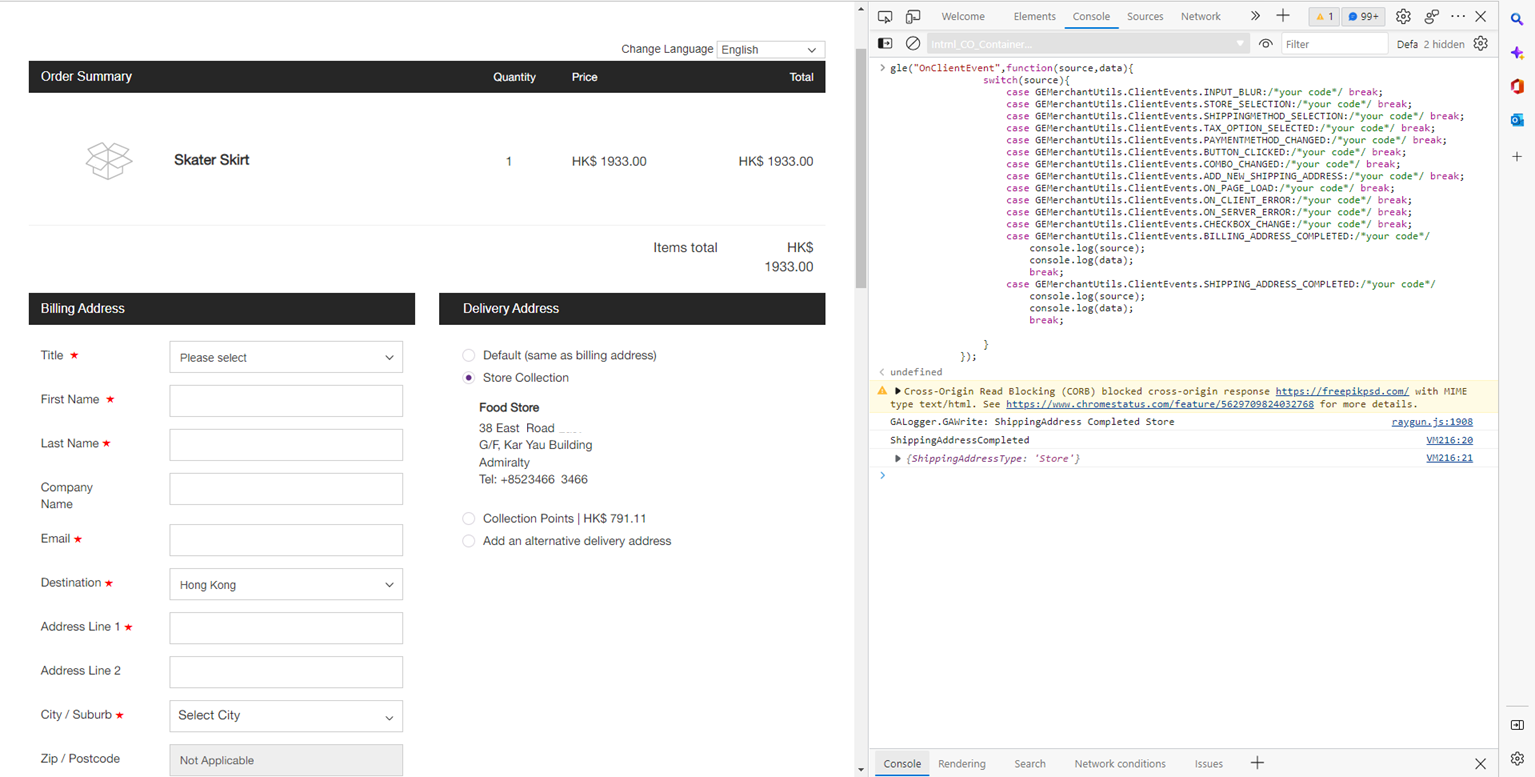
Example Code
gle("OnClientEvent",function(source,data){ switch(source){ case GEMerchantUtils.ClientEvents.INPUT_BLUR:/*your code*/ break; case GEMerchantUtils.ClientEvents.STORE_SELECTION:/*your code*/ break; case GEMerchantUtils.ClientEvents.SHIPPINGMETHOD_SELECTION:/*your code*/ break; case GEMerchantUtils.ClientEvents.TAX_OPTION_SELECTED:/*your code*/ break; case GEMerchantUtils.ClientEvents.PAYMENTMETHOD_CHANGED:/*your code*/ break; case GEMerchantUtils.ClientEvents.BUTTON_CLICKED:/*your code*/ break; case GEMerchantUtils.ClientEvents.COMBO_CHANGED:/*your code*/ break; case GEMerchantUtils.ClientEvents.ADD_NEW_SHIPPING_ADDRESS:/*your code*/ break; case GEMerchantUtils.ClientEvents.ON_PAGE_LOAD:/*your code*/ break; case GEMerchantUtils.ClientEvents.ON_CLIENT_ERROR:/*your code*/ break; case GEMerchantUtils.ClientEvents.ON_SERVER_ERROR:/*your code*/ break; case GEMerchantUtils.ClientEvents.CHECKBOX_CHANGE:/*your code*/ break; } });